Content modifier rule
TypeScript type: ContentModifier.
Content modifiers are used to modify how instances of specified ECClasses are displayed in content which is produced using content rules. They do not produce any content by themselves.
Attributes
Attribute: class
Specification of ECClass whose content should be modified. The modifier applies property overrides and property categories to all content if this attribute is not specified. This attribute must be specified in order to apply related or calculated properties to the content.
// The ruleset has a content rule that returns content of all `bis.SpatialCategory` and `bis.GeometricModel`
// instances.There's also a content modifier that creates a custom calculated property only for `bis.Category` instances.
const ruleset: Ruleset = {
id: "example",
rules: [
{
ruleType: "Content",
specifications: [
{
// load content for all `bis.SpatialCategory` and `bis.GeometricModel` instances
specType: "ContentInstancesOfSpecificClasses",
classes: { schemaName: "BisCore", classNames: ["SpatialCategory", "GeometricModel"], arePolymorphic: true },
},
],
},
{
ruleType: "ContentModifier",
class: { schemaName: "BisCore", className: "Category" },
calculatedProperties: [
{
label: "Calculated",
value: `"PREFIX_" & this.CodeValue`,
},
],
},
],
};
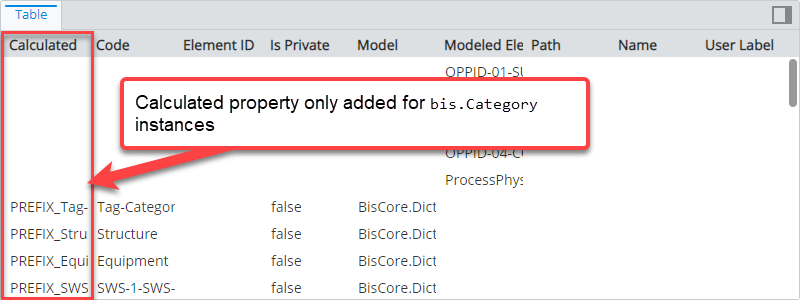
Attribute: requiredSchemas
A list of ECSchema requirements that need to be met for the rule to be used.
// The ruleset has a content rule that returns content of given input instances. There's also
// a content modifier that tells us to load `bis.ExternalSourceAspect` related properties, but the
// ECClass was introduced in BisCore version 1.0.2, so the modifier needs a `requiredSchemas` attribute
// to only use the rule if the version meets the requirement.
const ruleset: Ruleset = {
id: "example",
rules: [
{
ruleType: "Content",
specifications: [
{
// load content for given input instances
specType: "SelectedNodeInstances",
},
],
},
{
ruleType: "ContentModifier",
requiredSchemas: [{ name: "BisCore", minVersion: "1.0.2" }],
class: { schemaName: "BisCore", className: "ExternalSourceAspect" },
relatedProperties: [
{
// request to include properties of related ExternalSourceAspect instances
propertiesSource: {
relationship: { schemaName: "BisCore", className: "ElementOwnsMultiAspects" },
direction: "Forward",
targetClass: { schemaName: "BisCore", className: "ExternalSourceAspect" },
},
},
],
},
],
};
Attribute: priority
Defines the order in which rules are handled, higher number means the rule is handled first. If priorities are equal, the rules are handled in the order they're defined.
|
|
Type |
number |
Is Required |
No |
Default Value |
1000 |
// The ruleset has a content rule that returns content of all `bis.SpatialCategory`
// instances.There's also a content modifier that tells us to hide all properties
// of `bis.Element` instances and a higher priority modifier that tells us to show
// its `CodeValue` property.
const ruleset: Ruleset = {
id: "example",
rules: [
{
ruleType: "Content",
specifications: [
{
// load content of all `bis.SpatialCategory` instances
specType: "ContentInstancesOfSpecificClasses",
classes: { schemaName: "BisCore", classNames: ["SpatialCategory"], arePolymorphic: true },
},
],
},
{
ruleType: "ContentModifier",
class: { schemaName: "BisCore", className: "SpatialCategory" },
priority: 1,
propertyOverrides: [
{
// hide all properties
name: "*",
isDisplayed: false,
},
],
},
{
ruleType: "ContentModifier",
class: { schemaName: "BisCore", className: "SpatialCategory" },
priority: 2,
propertyOverrides: [
{
// display the CodeValue property
name: "CodeValue",
isDisplayed: true,
doNotHideOtherPropertiesOnDisplayOverride: true,
},
],
},
],
};
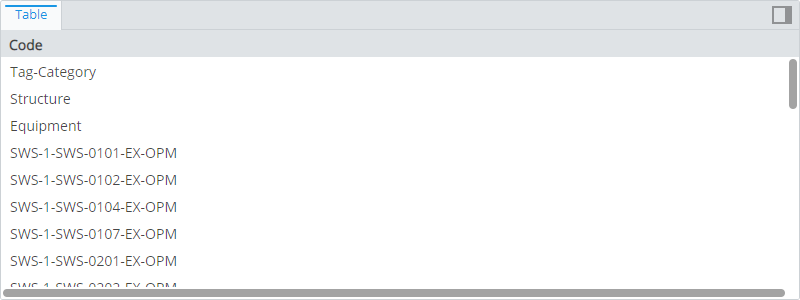
Attribute: applyOnNestedContent
Specifies whether calculatedProperties and relatedProperties specifications should also be applied on
nested content.
|
|
Type |
boolean |
Is Required |
No |
Default Value |
false |
// The ruleset has a content rule that returns content of given input instances. The produced content
// additionally include properties of parent element by following the `bis.SpatialViewDefinitionUsesModelSelector`
// relationship. There's also a content modifier that includes properties of the related `DisplayStyle` and
// creates a calculated property for nested `bis.SpatialViewDefinition`.
const ruleset: Ruleset = {
id: "example",
rules: [
{
ruleType: "Content",
specifications: [
{
// load content for given input instances
specType: "SelectedNodeInstances",
relatedProperties: [
{
propertiesSource: {
relationship: {
schemaName: "BisCore",
className: "SpatialViewDefinitionUsesModelSelector",
},
direction: "Backward",
},
},
],
},
],
},
{
// add a content modifier for `SpatialViewDefinition` elements
ruleType: "ContentModifier",
class: { schemaName: "BisCore", className: "SpatialViewDefinition" },
relatedProperties: [
{
propertiesSource: {
relationship: {
schemaName: "BisCore",
className: "ViewDefinitionUsesDisplayStyle",
},
direction: "Forward",
},
},
],
calculatedProperties: [
{
label: "Calculated for SpatialViewDefinition",
value: "this.Pitch",
},
],
// set `applyOnNestedContent: true` to get this modifier applied when `SpatialViewDefinition` content is loaded indirectly
applyOnNestedContent: true,
},
],
};
applyOnNestedContent: false |
applyOnNestedContent: true |
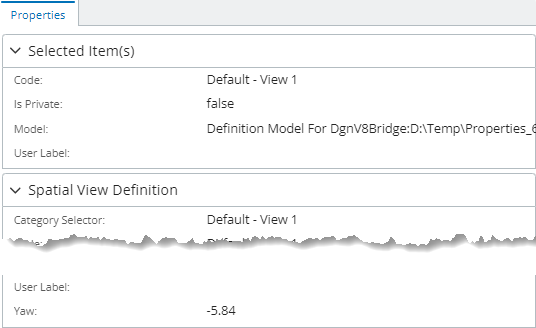 |
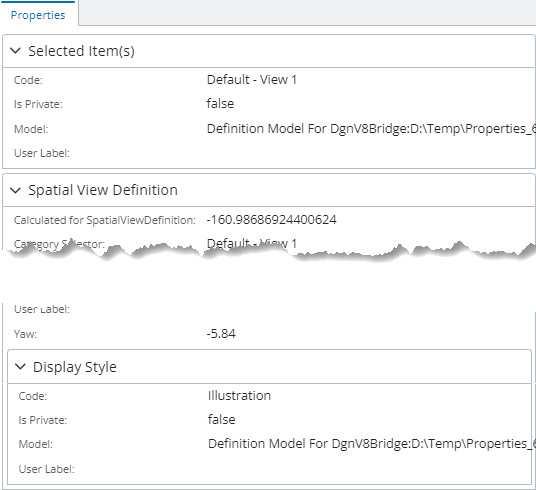 |
Attribute: relatedProperties
Specifications of related properties which are included in the generated content.
// The ruleset has a content rule that returns content of given input instances. There's also
// a content modifier that includes properties of the related `bis.Category` for all `bis.GeometricElement3d`
// instances' content.
const ruleset: Ruleset = {
id: "example",
rules: [
{
ruleType: "Content",
specifications: [
{
// load content for given input instances
specType: "SelectedNodeInstances",
},
],
},
{
ruleType: "ContentModifier",
class: { schemaName: "BisCore", className: "GeometricElement3d" },
relatedProperties: [
{
propertiesSource: {
relationship: { schemaName: "BisCore", className: "GeometricElement3dIsInCategory" },
direction: "Forward",
},
handleTargetClassPolymorphically: true,
},
],
},
],
};
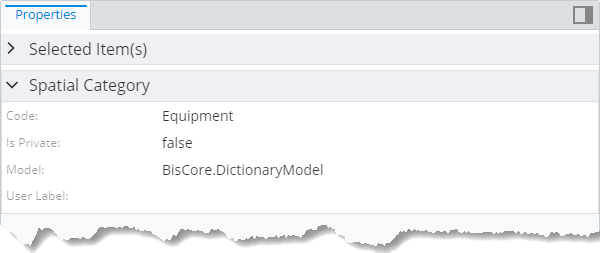
Attribute: calculatedProperties
Specifications of calculated properties whose values are generated using provided ECExpressions.
// The ruleset has a content rule that returns content of given input instances. There's also
// a content modifier that creates a calculated property for `bis.GeometricElement3d` instances.
const ruleset: Ruleset = {
id: "example",
rules: [
{
ruleType: "Content",
specifications: [
{
// load content for given input instances
specType: "SelectedNodeInstances",
},
],
},
{
ruleType: "ContentModifier",
class: { schemaName: "BisCore", className: "GeometricElement3d" },
calculatedProperties: [
{
label: "Yaw & Pitch & Roll",
value: `this.Yaw & " & " & this.Pitch & " & " & this.Roll`,
},
],
},
],
};
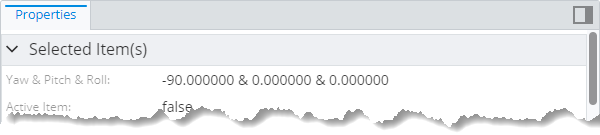
Attribute: propertyCategories
Specifications for custom categories. Simply defining the categories does nothing - they have to be referenced from property specification defined in propertyOverrides by id
.
// The ruleset has a content rule that returns content of given input instances. There's also
// a content modifier that moves all `bis.GeometricElement3d` properties into a custom category.
const ruleset: Ruleset = {
id: "example",
rules: [
{
ruleType: "Content",
specifications: [
{
// load content for given input instances
specType: "SelectedNodeInstances",
},
],
},
{
ruleType: "ContentModifier",
class: { schemaName: "BisCore", className: "GeometricElement3d" },
propertyCategories: [
{
id: "custom-category",
label: "Custom Category",
},
],
propertyOverrides: [
{
name: "*",
categoryId: "custom-category",
},
],
},
],
};
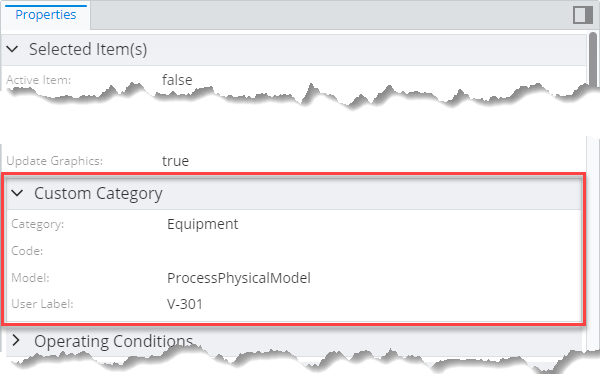
Attribute: propertyOverrides
Specifications for various property overrides that allow customizing property display.
// The ruleset has a content rule that returns content of given input instances. There's also
// a content modifier that customizes display of `bis.GeometricElement3d` properties.
const ruleset: Ruleset = {
id: "example",
rules: [
{
ruleType: "Content",
specifications: [
{
// load content for given input instances
specType: "SelectedNodeInstances",
},
],
},
{
ruleType: "ContentModifier",
class: { schemaName: "BisCore", className: "GeometricElement3d" },
propertyOverrides: [
{
// force hide the UserLabel property
name: "UserLabel",
isDisplayed: false,
},
{
// force show the Parent property which is hidden by default through ECSchema
name: "Parent",
isDisplayed: true,
doNotHideOtherPropertiesOnDisplayOverride: true,
},
{
// override label of CodeValue property
name: "CodeValue",
labelOverride: "Overriden Label",
},
],
},
],
};
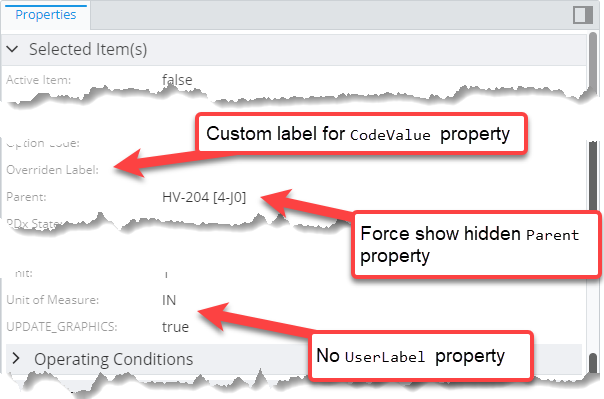